모바일 웹 사이트와 스마트 폰, 태블릿 및 데스크톱 응용 프로그램을 만들 수 있도록 설계된 HTML5 기반 프레임워크다.
이번에는 화면 왼쪽에서 편리하게 사용할 수 있는 패널과 JSON 데이터를 읽어 목록에 추가하는 예제는 기본 템플릿에서 부터 만들어 보자.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>jQuery Mobile</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css" />
<script src="http://code.jquery.com/jquery-1.11.1.min.js"></script>
<script src="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script>
</head>
<body>
<section id="page1" data-role="page" data-add-back-btn="true" data-theme="b" data-rel="back">
<header data-role="header" data-position="fixed">
<h1>헤더</h1>
</header>
<div data-role="content">
<h1>본문</h1>
</div>
<footer data-role="footer" data-position="fixed">
<h2>주한길</h2>
</footer>
</section>
</body>
</html>
처음 로딩 화면 (기본 템플릿)
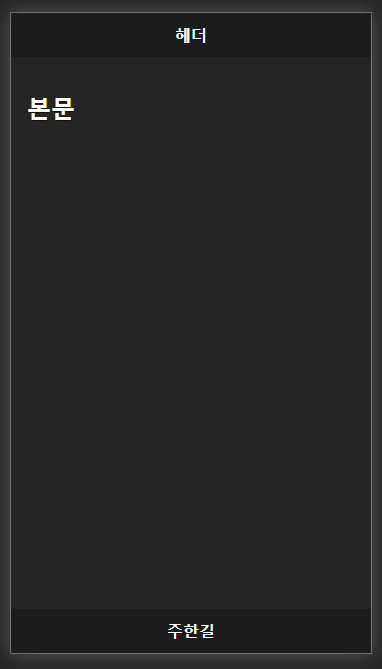
<section> 태그와 <header> 태그 사이에 패널 UI를 생성하고 헤더에서 패널을 열기 위한 버튼을 하나 생성한다.
<section id="page1" data-role="page" data-add-back-btn="true" data-theme="b" data-rel="back">
<div data-role="panel" id="myPanel" data-display="push">
<h2>패널</h2>
<p>패널 밖을 누르거나 아래의 버튼을 클릭하세요.</p>
<a href="#" data-rel="close"
class="ui-btn ui-btn-inline ui-icon-recycle ui-btn-icon-left ui-shadow data-theme="b">패널 닫기</a>
</div>
<header data-role="header" data-position="fixed">
<a href="#myPanel" class="ui-btn-left" data-icon="back">패널 열기</a>
<h1>헤더</h1>
</header>
... (중략) ...
패널 열기 버튼을 클릭하여 패널 열기
패널 밖을 누르거나 아래의 버튼을 클릭하면 패널이 사라진다.
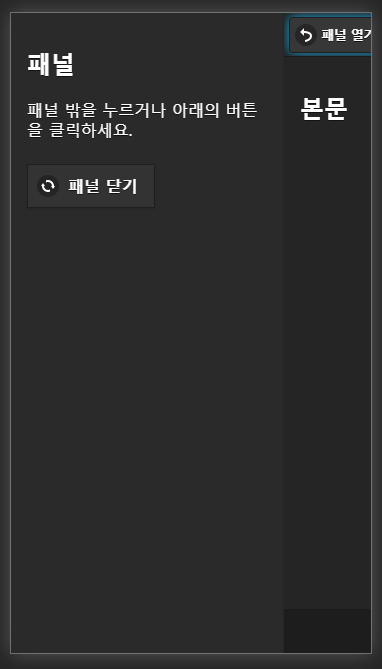
본문에 다음과 같이 <ul>태그로 구성된 리스트뷰를 하나 만들어 둔다. ID는 "listPlant"이다.
<div data-role="content">
<h1>PLANT</h1>
<ul id="listPlant" data-role="listview" data-inset="true">
</ul>
</div>
listPlant 리스트뷰에 목록을 표시하기 위해 jQuery코드를 추가한다.
<script>
$(function() {
var plantList = [ {
"code" : "KOR01",
"name" : "기흥",
}, {
"code" : "KOR02",
"name" : "수원",
}, {
"code" : "KOR03",
"name" : "천안",
}, {
"code" : "CHN01",
"name" : "텐진",
}, {
"code" : "CHN02",
"name" : "선전",
} ];
var output = '';
$.each(plantList, function(i, plant) {
output += '<li id=' + plant.code +'>';
output += '<a href="#">' + (i + 1) + ". " + plant.code + ' - ' + plant.name + '</a></li>';
});
$('#listPlant').html(output).listview("refresh");
})
</script>
데이터가 리스트뷰에 표시
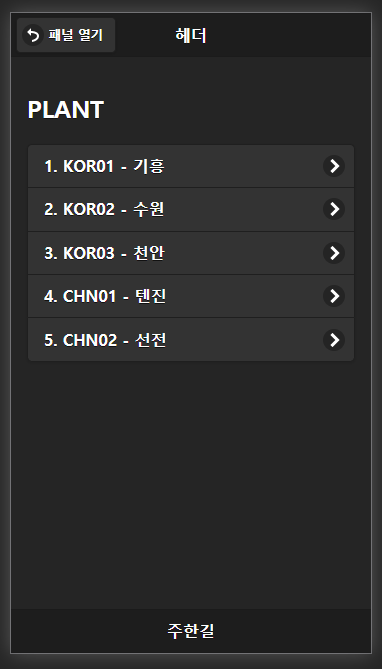
다음과 같이 footer에 표시할 기능 버튼을 추가한다.
<footer data-role="footer" data-position="fixed" style="text-align: center;">
<div data-role="controlgroup" data-type="horizontal">
<a href="#" class="ui-btn ui-icon-plus ui-btn-icon-left">추가</a>
<a href="#" class="ui-btn ui-icon-check ui-btn-icon-left">수정</a>
<a href="#" class="ui-btn ui-icon-minus ui-btn-icon-left">삭제</a>
<a href="#" class="ui-btn ui-icon-refresh ui-btn-icon-left">새로코침</a>
</div>
</footer>
전체 소스코드
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>jQuery Mobile</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.css" />
<script src="http://code.jquery.com/jquery-1.11.1.min.js"></script>
<script src="http://code.jquery.com/mobile/1.4.5/jquery.mobile-1.4.5.min.js"></script>
<script>
$(function() {
var plantList = [ {
"code" : "KOR01",
"name" : "기흥",
}, {
"code" : "KOR02",
"name" : "수원",
}, {
"code" : "KOR03",
"name" : "천안",
}, {
"code" : "CHN01",
"name" : "텐진",
}, {
"code" : "CHN02",
"name" : "선전",
} ];
var output = '';
$.each(plantList, function(i, plant) {
output += '<li id=' + plant.code +'>';
output += '<a href="#">' + (i + 1) + ". " + plant.code + ' - ' + plant.name + '</a></li>';
});
$('#listPlant').html(output).listview("refresh");
})
</script>
</head>
<body>
<section id="page1" data-role="page" data-add-back-btn="true" data-theme="b" data-rel="back">
<div data-role="panel" id="myPanel" data-display="push">
<h2>패널</h2>
<p>패널 밖을 누르거나 아래의 버튼을 클릭하세요.</p>
<a href="#" data-rel="close"
class="ui-btn ui-btn-inline ui-icon-recycle ui-btn-icon-left ui-shadow data-theme="b">패널 닫기</a>
</div>
<header data-role="header" data-position="fixed">
<a href="#myPanel" class="ui-btn-left" data-icon="back">패널 열기</a>
<h1>패널과 리스트</h1>
</header>
<div data-role="content">
<h1>PLANT</h1>
<ul id="listPlant" data-role="listview" data-inset="true">
</ul>
</div>
<footer data-role="footer" data-position="fixed" style="text-align: center;">
<div data-role="controlgroup" data-type="horizontal">
<a href="#" class="ui-btn ui-icon-plus ui-btn-icon-left">추가</a>
<a href="#" class="ui-btn ui-icon-check ui-btn-icon-left">수정</a>
<a href="#" class="ui-btn ui-icon-minus ui-btn-icon-left">삭제</a>
<a href="#" class="ui-btn ui-icon-refresh ui-btn-icon-left">새로코침</a>
</div>
</footer>
</section>
</body>
</html>
jqm_17.html
0.00MB
완성된 화면
728x90
'■ Data Skill ■ > jQuery Mobile' 카테고리의 다른 글
[jQuery 모바일] 목록 테이블과 컬럼표시 연동 (0) | 2023.02.11 |
---|---|
[jQuery 모바일] 펼치기,접기와 몇가지팁 (0) | 2023.02.11 |
[jQuery 모바일] 여러가지 버튼과 리스트 (0) | 2023.02.11 |
[jQuery 모바일] 여러가지 선택항목 (Select, Radio, Checkbox) (0) | 2023.02.11 |
[jQuery 모바일] 여러가지 다양한 항목들 (0) | 2023.02.11 |
댓글